새로운 Activity를 띄우지 않고 Windows의 팝업 창처럼 그냥 창을 띄워 상태 알람/진행을 표시하는 녀석이다.
아래 Sample은 각각 종류별로 정리했다. 종류는 아래와 같다.
- Basic Alert - 아주 기본적인 Alert Dialog
- Button add - Dialog 창에 Button이 추가
- List type - 여러가지 항목을 선택
- CheckBox type - CheckBox가 포함
- RadioButton type - RadioButton이 포함(미리 체크 항목을 선택 할 수 있음)
- ProgressDialog type - 동그란 에니메이션이 동작함
- ProgressBar type - 막대 형태로 진행 상태를 표시함
- Custom Dialog - 임의 디자인을 적용
1. Basic Alert
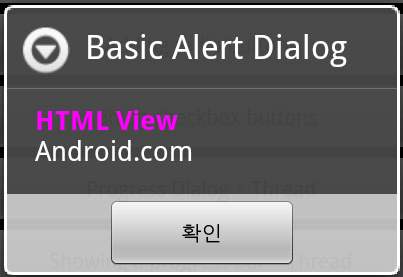
AlertDialog.Builder ab = null; ab = new AlertDialog.Builder( AlertDialogTest.this ); ab.setMessage( Html.fromHtml("<b><font color=#ff00ff> HTML View</font></b><br>Android.com")); ab.setPositiveButton(android.R.string.ok, null); ab.setTitle( "Basic Alert Dialog" ); ab.show();
|
2. Button add
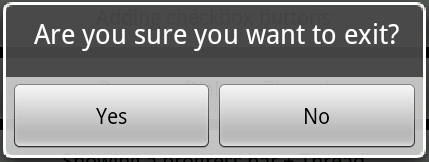
AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setMessage("Are you sure you want to exit?") .setCancelable(false) .setPositiveButton("Yes", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(getApplicationContext(), "ID value is " + Integer.toString(id), Toast.LENGTH_SHORT).show(); } }) .setNegativeButton("No", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int id) { Toast.makeText(getApplicationContext(), "ID value is " + Integer.toString(id), Toast.LENGTH_SHORT).show(); dialog.cancel(); } });
AlertDialog alert = builder.create(); alert.show();
|
3. List type
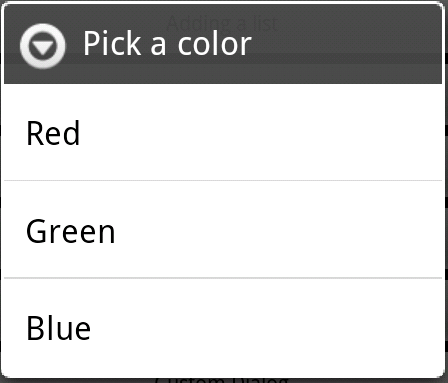
final CharSequence[] items = {"Red", "Green", "Blue"};
AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Pick a color"); builder.setItems(items, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int item) { Toast.makeText(getApplicationContext(), items[item], Toast.LENGTH_SHORT).show(); } }); AlertDialog alert = builder.create(); alert.show();
|
4. RadioButtons type
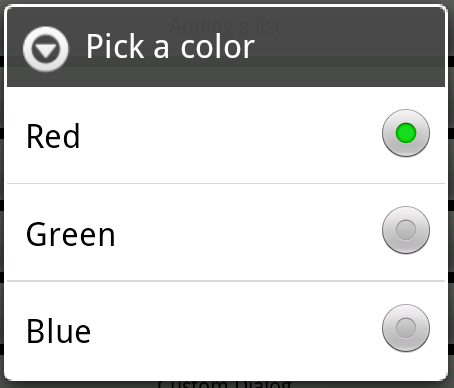
final CharSequence[] items = {"Red", "Green", "Blue"};
AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Pick a color"); builder.setSingleChoiceItems(items, -1, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int item) { Toast.makeText(getApplicationContext(), items[item], Toast.LENGTH_SHORT).show(); } }); AlertDialog alert = builder.create(); alert.show();
|
5. Checkboxes type
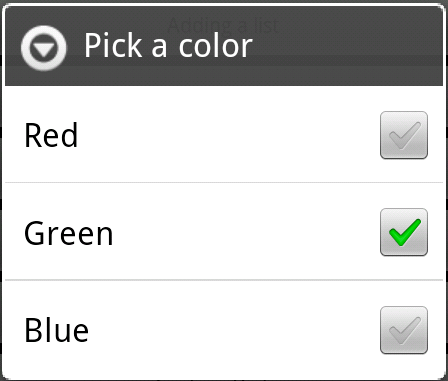
final CharSequence[] items = {"Red", "Green", "Blue"}; final boolean[] itemsChecked = {false, true, false };
AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Pick a color"); builder.setMultiChoiceItems( items, itemsChecked, new DialogInterface.OnMultiChoiceClickListener() {
@Override public void onClick(DialogInterface dialog, int which, boolean isChecked) { Toast.makeText( getApplicationContext(), items[which] + " Checked-" + Boolean.toString(isChecked), Toast.LENGTH_SHORT).show(); } });
AlertDialog alert = builder.create(); alert.show();
|
6. ProgressDialog type
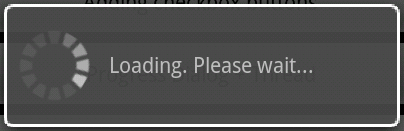
private class ProgressDialogStop extends Thread { private final int mWaitTime = 3000; private ProgressDialog dialog = null; public ProgressDialogStop(ProgressDialog dialog) { // TODO Auto-generated constructor stub this.dialog = dialog; } @Override public void run() { int iWait = 0; try { while( iWait < mWaitTime ) { this.sleep(100); iWait += 100; } }catch(Exception e) { // } this.dialog.dismiss(); super.run(); } }
|
ProgressDialog dialog = ProgressDialog.show(AlertDialogTest.this, "", "Loading. Please wait...", true);
Thread ProgressDialogStopThread = new ProgressDialogStop( dialog ); ProgressDialogStopThread.start();
|
7. ProgressBar type
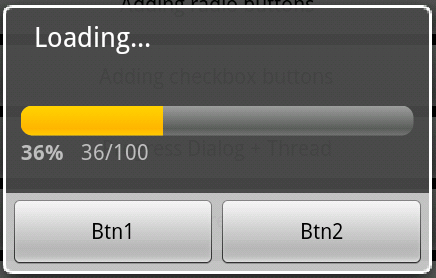
private class ProgressBarStop extends Thread { private final int mWaitTime = 100; private ProgressDialog progressDialog = null; public ProgressBarStop(ProgressDialog progressDialog) { // TODO Auto-generated constructor stub this.progressDialog = progressDialog; } @Override public void run() { super.run(); // this.progressDialog.show(); // << 여기서 호출하면 Error 발생한다. int iWait = 0; try { while( iWait < mWaitTime ) { this.sleep(100); iWait++; Log.i(LOGTAG, Integer.toString(iWait)); progressDialog.setProgress( iWait ); } }catch(Exception e) { Log.i(LOGTAG, e.getMessage()); } this.progressDialog.dismiss(); } }
|
ProgressDialog progressDialog = null; progressDialog = new ProgressDialog( AlertDialogTest.this ); progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL); progressDialog.setMessage("Loading..."); progressDialog.setMax(100); progressDialog.setCancelable(true);
progressDialog.setButton("Btn1", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { Toast.makeText( getApplicationContext(), "Btn Click - " + Integer.toString(which), Toast.LENGTH_SHORT).show(); } });
progressDialog.setButton2("Btn2", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { Toast.makeText( getApplicationContext(), "Btn 2 Click - " + Integer.toString(which), Toast.LENGTH_SHORT).show(); } });
progressDialog.show(); Thread PrgrsBarStop = new ProgressBarStop( progressDialog ); PrgrsBarStop.start();
|
8. CustomDialog type
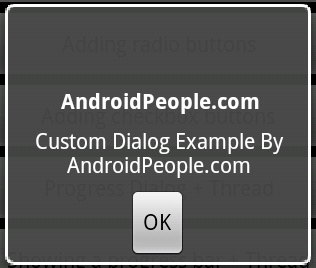
별도 Dialog 타입 Layout을 설정해야 한다.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/LinearLayout01" android:layout_height="fill_parent" android:orientation="vertical" android:gravity="center" android:layout_width="200dip"> <TextView android:id="@+id/TextView01" android:layout_height="wrap_content" android:textColor="#fff" android:textStyle="bold" android:layout_width="wrap_content" android:text=" AndroidPeople.com"/> <TextView android:id="@+id/TextView02" android:layout_height="wrap_content" android:textColor="#fff" android:layout_width="wrap_content" android:layout_margin="7dip" android:text="Custom Dialog Example By AndroidPeople.com" android:gravity="center"/> <Button android:id="@+id/OkButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="OK" /> </LinearLayout>
|
import android.app.Dialog; import android.content.Context; import android.os.Bundle; import android.view.View; import android.widget.Button; //http://about-android.blogspot.com/2010/02/create-custom-dialog.html public class MyCustomDialog extends Dialog {
public MyCustomDialog(Context context) { super(context); this.setContentView(R.layout.custom_dialog); } @Override protected void onCreate(Bundle savedInstanceState) { // TODO Auto-generated method stub super.onCreate(savedInstanceState); Button btn = (Button)findViewById(R.id.OkButton); btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { dismiss(); } }); } }
|
Dialog dialog = new MyCustomDialog(this);
dialog.show();
|
9. CustomDialog type 2
위 8번 내용을 그대로 사용.
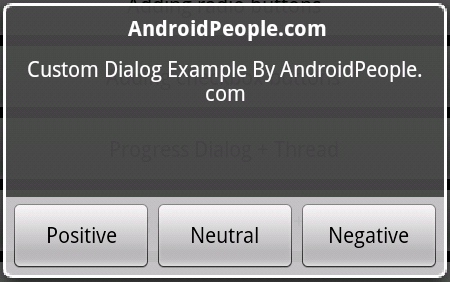
AlertDialog.Builder md = new AlertDialog.Builder(this); LayoutInflater contents = LayoutInflater.from(this); View customView = contents.inflate(R.layout.custom_dialog, null);
Button btn = (Button)customView.findViewById(R.id.OkButton); btn.setVisibility(View.INVISIBLE);
md.setPositiveButton("Positive", new DialogInterface.OnClickListener() {
@Override public void onClick(DialogInterface dialog, int which) { } });
md.setNegativeButton("Negative", new DialogInterface.OnClickListener() {
@Override public void onClick(DialogInterface dialog, int which) { } });
md.setNeutralButton("Neutral", new DialogInterface.OnClickListener() {
@Override public void onClick(DialogInterface dialog, int which) { } });
md.setView( customView ); md.show();
|
출처 : http://utime.blog.me/150091024547